Cadence
What is Cadence
Cadence is a resource-oriented, high-level programming language designed for developing smart contracts. It prioritizes safety, readability, and ease of use, making it an excellent choice for developers looking to build secure and scalable blockchain applications.
Cadence introduces a novel approach to smart contract development, leveraging strong static typing, capability-based security, and an intuitive syntax inspired by Swift and Rust. With built-in protections against common vulnerabilities like reentrancy attacks and unauthorized asset transfers, Cadence ensures that smart contracts remain secure and predictable.
Think of Cadence as the next-gen language for creating powerful onchain logic and seamless experience apps — without compromising security or composability.
Why Cadence?
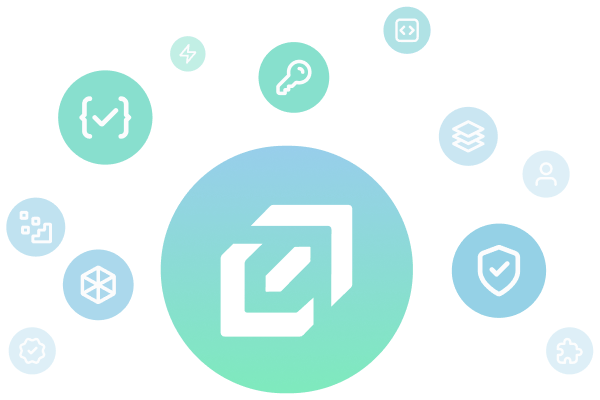
Powerful Features Built for Web3 Developers
Core Language Concepts
// Object users store in their account, represents currency
resource Vault {
access(all) var balance: UFix64
init(balance: UFix64) {
self.balance = balance
}
}
let vault <- create Vault(balance: 10.0) // creation
let myVault <- vault // move with <-
vault.balance // Invalid: Cannot access: The old vault was moved
let vaultCopy = myVault // Invalid: Cannot make copies of resources
let name: String = "Cadence"
// Invalid: cannot assign an `Int` value to a `String` variable.
//
name = 0
// Language infers the type to be `Int` without a specification
let age = 32
// Object users store in their account, represents currency
resource Vault {
access(all) var balance: UFix64
// Only those with a `Withdraw` reference to this object
// can call this function. Only the owner can grant a Withdraw reference
access(Withdraw) fun withdraw(amount: UFix64)
}
// Create a private withdraw capability in storage that
// can only be given to those you trust
withdrawCapability = account.capabilities.issue<auth(Withdraw) &Vault>(
target: /storage/myVault
)
// Create a public capability that allows anyone to deposit currency
// to your account because it is created in a `public` path
signer.capabilities.publish (
signer.capabilities.storage.issue<&Vault>(
vaultData.storagePath
),
at: /public/vaultReceiver
)
// Declare an interface that requires the`greet` function
access(all) contract interface Greeter {
access(all) fun greet(): String
}
// A contract can implement interfaces to enforce correctness of implementation
access(all) contract HelloWorld: Greeter {
access(all) fun greet(): String {
return "Hello, Flow!"
}
}
// Interfaces can also be used for composite types!
access(all) resource interface Balance {
access(all) var balance: UFix64
}
access(all) fun withdraw(amount: UFix64): @Vault {
pre {
amount <= self.balance: "Amount to withdraw cannot be more than the balance!"
}
post {
result.balance == before(result.balance) - amount: "Wrong amount withdrawn!"
}
self.balance = self.balance - amount
return <-create Vault(balance: amount)
}
Advanced Features
access(all) view fun sayHello(name: String): String {
return "Hello, \(name)"
}
access(all) event SignatureVerified(publicKey: String, signature: String)
let pk = PublicKey(
publicKey: "96142CE0C5ECD869DC88C8960E286AF1CE1B29F329BA4964213934731E65A1DE480FD43EF123B9633F0A90434C6ACE0A98BB9A999231DB3F477F9D3623A6A4ED".decodeHex(),
signatureAlgorithm: SignatureAlgorithm.ECDSA_P256
)
let signature = "108EF718F153CFDC516D8040ABF2C8CC7AECF37C6F6EF357C31DFE1F7AC79C9D0145D1A2F08A48F1A2489A84C725D6A7AB3E842D9DC5F8FE8E659FFF5982310D".decodeHex()
let message : [UInt8] = [1, 2, 3]
let isValid = pk.verify(
signature: signature,
signedData: message,
domainSeparationTag: "",
hashAlgorithm: HashAlgorithm.SHA2_256
)
if isValid { emit SignatureVerified(publicKey: publicKey, signature: signature)
transaction {
// Authorize with multiple accounts
// Only one needs to be the payer
prepare(acct1: &Account, acct2: &Account) {
log("Acquire things from both authorizing accounts' storage")
}
execute {
// Call multiple contracts in a single transaction
FooContract.foo()
BarContract.bar()
}
post {
FooContract.callCount == before(FooContract.callCount + 1):
"Post-assertions must pass or the whole transaction reverts"
}
}
// Standard way for a project to represent its basic metadata
access(all) struct Display {
access(all) let name: String
access(all) let description: Address
access(all) let thumbnail: {File}
}
// Standard way for a resource object
// to return arbitrary metadata views that it supports
access(all) resource interface Resolver {
// Caller specifies which type of metadata it is looking for
// and the function returns the relevant representation
access(all) fun resolveView(_ view: Type): AnyStruct?
}
access(all) attachment Skin for GamePiece.NFT {
access(all) let skinType: String
init(_ skinType: String) {
self.skinType = skinType
}
}
access(all) fun addAttachmentTo(
nft: @GamePiece.NFT,
skinType: String
): @GamePiece.NFT {
if nft[Skin] != nil {
// Skin attachment already attached - return
return <- nft
}
// Attach the Skin & return
return <- attach Skin(skinType) to <- nft
}